LWC Interview Questions Part-1
Why LWC:
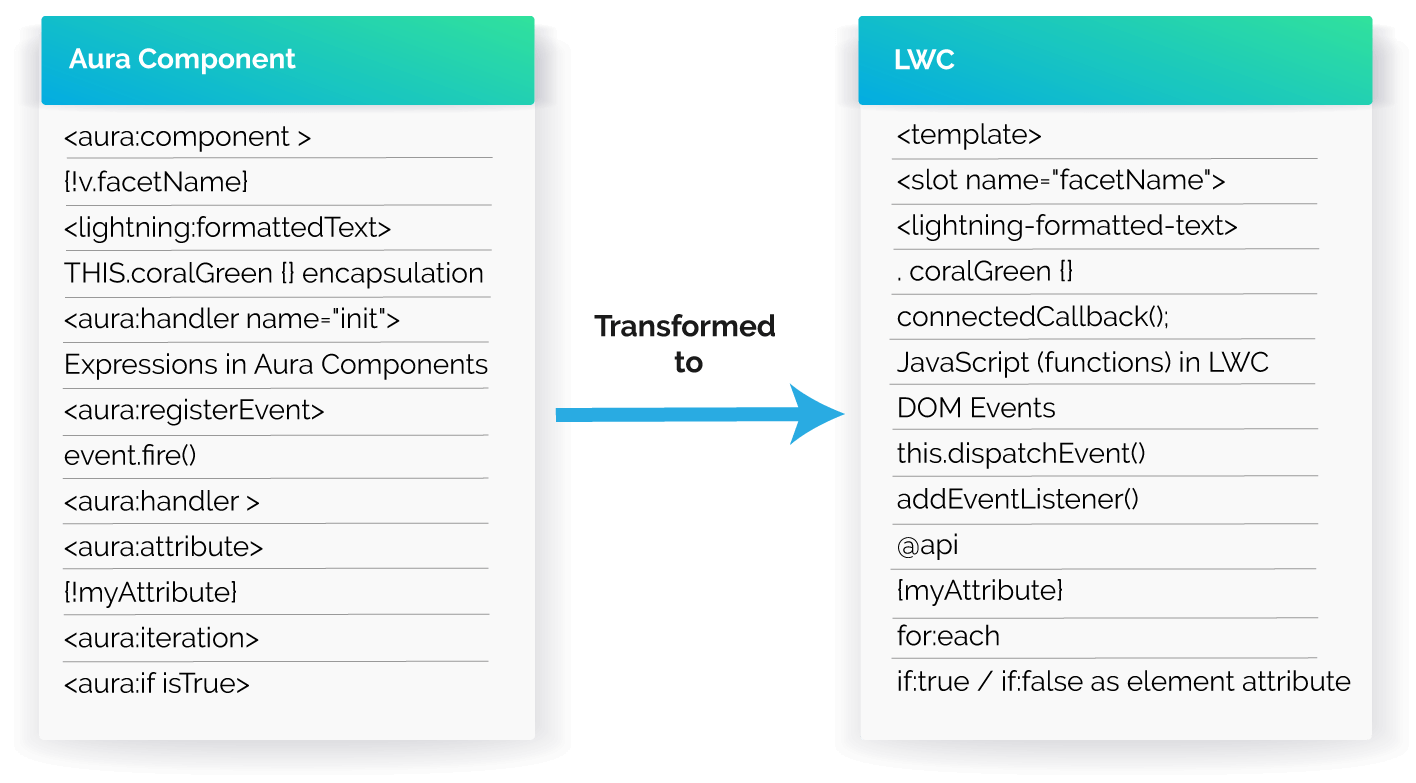
The first thing we will know about what web components are. Web Components consist of four separate technologies that are used together:
- **Custom Elements:**The HTML elements with custom templates, tag names like and behaviours are made with a set of JavaScript APIs. HTML Living Standard specification has definitions of these Custom Elements.
- **Shadow DOM:**We use this for isolating CSS and JavaScript which could be like. Living Standard DOM specification has the definitions of this.
- **HTML templates:**User-defined templates in HTML are rendered only when called upon. HTML Living Standard specification has the definition of the tag.
- **ES Modules:**The ES Modules specification defines the inclusion and reuse of JS documents in a standard-based, modular, performant way.
Usage of Modern JavaScript
Why do you go for LWC instead of existing Aura Components?
- Easy to Learn: LWC is basically taking the form through native web standards that is in the browser. It means that no added abstraction layer like Aura Framework or any other framework, we only need standard JavaScript to develop.
- Better Performance: Because of the no added abstraction layer, LWC is likely to render faster than aura components since performance is important to deliverability.
- Faster loading sites: Like lightning, LWC is faster in loading the developed components than Aura Components and is a lightweight framework that is built on web standards.
- More Standards, less proprietary: LWC has built-in browser security features from Web Components Standards, so the usage of out-of-the-box is more and less of customization. We all know that Aura is proprietary so with LWC, the more we know about web standards; more we’ll have the skill that can be used in other technologies as well.
- Common Components/Service Components: We can now write components that have no User Interface in LWC, and those components can be reused in other components that are more efficient than Static Resources.
- Easier to ramp for developers: No additional framework is needed to learn in order to develop LWC and hence transition for the developers is a lot easier.
- Better security, better testing, and better browser compatibility: With LWC, CSS, Script, and DOM Isolation are better and has more limited event scope. With each of these, we have more consistency in designing Components. LWC also supports Two-way data binding with which we can coordinate how data moves between Components
We all know about Lightning Data Service (LDS) in Lightning Components. It is basically used to create, update, read, and delete a record without any using Apex Code. Similarly, LWC can also leverage LDS by accessing data and metadata from all Standard and Custom Objects.
Import from ‘lightning/uiRecordApi’
- createRecord(recordInput)
- updateRecord(recordInput)
- deleteCreateRecord(recordInput)
- getRecord(recordInput)
Interoperability between Lightning Web Components and Lightning Components
- Aura and LWC can be able to communicate using Public API’s and Events.
- LWC can be embedded inside Aura Components, but Aura Components cannot be embedded inside LWC.
- To dig deep into this topic, Salesforce has provided a great article on this Interoperability.
Once we create a new lightning web component then by default 3 files get created:
.html : The power of Lightning Web Components is the templating system, which uses the virtual DOM to render components smartly and efficiently. It’s a best practice to let LWC manipulate the DOM instead of writing JavaScript to do it.
.js :
.js-meta.xml :
- HTML provides the structure for your component.
- JavaScript defines the core business logic and event handling.
- CSS provides the look, feel, and animation for your component.
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata" fqn="helloWorld">
<apiVersion>45.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
<target>lightning__RecordPage</target>
<target>lightning__HomePage</target>
</targets>
</LightningComponentBundle>
Example for 'IF' statement in LWC:
HTML:
.html
<template>
<div id="waiting" if:false={ready}>Loading…</div>
<div id="display" if:true={ready}>
<div>Name: {name}</div>
<div>Description: {description}</div>
<lightning-badge label={material}></lightning-badge>
<lightning-badge label={category}></lightning-badge> <!-- Display Badge-->
<div>Price: {price}</div>
<div><img src={pictureUrl}/></div>
</div>
</template>
.js
import { LightningElement } from 'lwc';
export default class App extends LightningElement {
name = 'Electra X4';
description = 'A sweet bike built for comfort.';
category = 'Mountain';
material = 'Steel';
price = '$2,700';
pictureUrl = '<https://s3-us-west-1.amazonaws.com/sfdc-demo/ebikes/electrax4.jpg>';
ready = false;
connectedCallback() {
setTimeout(() => {
this.ready = true;
}, 3000);
}
}
result:The export statement defines a class that extends the LightningElement class. As a best practice, the name of the class usually matches the file name of the JavaScript class, but it’s not a requirement.
What is need for import Keyword in JS:
The import statement indicates the JavaScript uses the LightningElement functionality from the lwc module.
-
LightningElement is the base class for Lightning web components, which allows us to use .
connectedCallback()
-
The method is one of our lifecycle hooks. You’ll learn more about lifecycle hooks in the next section. For now, know that the method is triggered when a component is inserted in the document object model (DOM). In this case, it starts the timer.
connectedCallback()
// import module elements
import { LightningElement} from 'lwc';
// declare class to expose the component
export default class App extends LightningElement {
ready = false;
// use lifecycle hook
connectedCallback() {
setTimeout(() => {
this.ready = true;
}, 3000);
}
}
//In the JavaScript file we used to test our conditional rendering, we used the connectedCallback() method to automatically execute code when the component is inserted into the DOM. The code waits 3 seconds, then sets ready to true
What is Decorator in LWC:Decorators are often used in JavaScript to modify the behavior of a property or function.
To use a decorator, import it from the lwc module and place it before the property or function.
Types of Decorator:
-api:
Make a field as public. All public properties are reactive, which means that the framework observes the property for changes. When the property’s value changes, the framework reacts and rerenders the component.-track:
Let us understand how to use the Tracked Properties in Lightning web components. To track a private property’s value and re-render a component when it changes, decorate the property with @track.Tracked properties are also called private reactive properties. Modern JavaScript framework is supporting “reactive,” properties which mean it can automatically refresh your data when changes have happened to the property and what does mean “private reactive “.private reactive means the data changes are atomically refreshing on the UI and not exposed to the other components or an app builder.These properties are strictly inside the component. Now let us understand the ways you can take advantage of this in your apps. You can use a tracked property directly in a template. You can also use a tracked property indirectly in a getter of a property that’s used in a template-wire :
Gives you an easy way to get and bind data from a Salesforce orgHandle Events in Lightning Web Components:
Handle Events:
Handle Events
Event propagation in LWC:
https://developer.salesforce.com/docs/component-library/documentation/en/lwc/lwc.events_propagation
Shadow DOM
Shadow DOM is a common implementation that allows a component’s elements to live in a “sub tree” of the DOM.
* More on Shadow Dom:https://bitsofco.de/what-is-the-shadow-dom/
Comments
Post a Comment